CSS: absolute basics
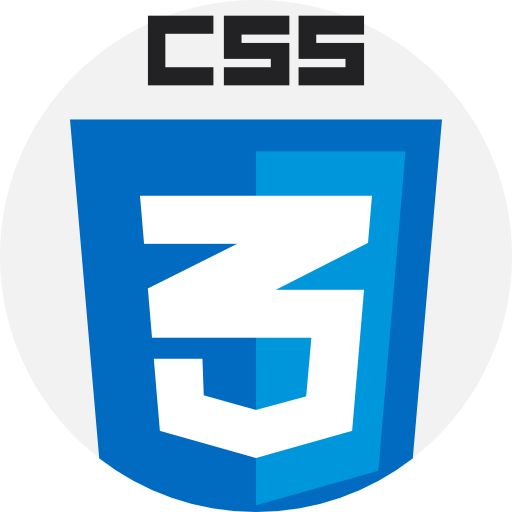
We start this CSS learning journey by introducing the basics you must know beofre going further. As usual with the articles on this blog, the focus is on the code with insightful comments instead of long and boring explanations.
In this article series, I assume that you are not a stranger to software development and you have an idea of what CSS is. You came here to find a concise presentation of CSS features that you can quickly pick and use in-code. For in-depth explanations, do not hesitate to consult mdn.
Base syntax
/* selector { property:value; property: value; ... } */
h1 { color: red; }
Selectors
Selector | Description |
---|---|
Universal: * |
Matches every single element on the page |
Type: p |
Matches every <p> element on the page |
Id: #cmpId |
Matches every element where id="cmpId" on the page |
Class: .cmpClass |
Matches every element where class="cmpClass" on the page |
Descendants: ul.nav li a |
Matches every element <a> inside an <li> inside a <ul> where class="nav" on the page |
Child: ul > li ol |
Matches every element <ol> inside an <li> of <ul> elements |
Adjacent siblings: ul.sports + p |
Matches every element <p> appearing after a <ul> at the same hierarchical level where class="sports" |
General siblings: ul.sports ~ p |
Matches every element <p> appearing before or after a <ul> at the same hierarchical level where class="sports" |
Pseudo-selectors
Pseudo-selectors let you target the states of an element to adapt its style dynamically depending on user interactions. A pseudo-selector can be target using the following syntax :
/* selector:pseudo-selector { property:value; property: value; ... } */
button:hover {
color: blue;
}
Common examples are :hover
, :first-child
and :last-childs
(in lists), :focus
, nth-child(n)
(tables or lists) ...
Pseudo-elements
Pseudo-elements allow you to select parts/areas of an element that do not have an ID or a class. It is helpful when you want to style the first letter of a paragraph or when you want to insert some content before/afer the element.
/* selector::pseudo-element { property:value; property: value; ... } */
p::first-line {
color: blue;
}
::before and :: after
::before
and ::after
are common pseudo-selectors used to decorate an element with rich content that is not part of the page's actual markup. You can inject strings, images or other resources using them.
h1::before{
content: url("img/bullet.png");
}
Attributes
You can match elements by their attributes as well, by either checking the attribute existence or its value. This is particularly useleful for styling forms.
/* selector[attrbiute expression] { property:value; property: value; ... } */
/* matches all elements that have a 'name' attribute */
[name] {
color: blue;
}
/* matches input elements of type 'password' */
input[type="password"] {
background-color: gray;
}
/* matches div elements where one of the attributes is 'navbar' */
div[type~="navbar"] {
background-color: gray;
}
/* matches a elements where the 'href' attributes starts with a particular pattern */
a[href^="ftp://"] {
background-color: gray;
}
/* matches a elements where the 'href' attributes ends with a particular pattern */
a[href$=".docx"] {
background-color: gray;
}
/* matches p elements where the 'class' attributes contains a particular pattern */
p[class*="intr"] {
background-color: gray;
}
Medias
The formatting of a web page is usually made to render on a screen but CSS supports several other types. The targeted device is called media
and you can adjust the styling of a page depending on the media that will render it.
Most common medias are screen
, print
(for printers when printing a page) and all
.
To adapt the styling to each media, simply tag the css entries with @media
:
@media screen {
h1 {
font-size: 14px;
font-family: Arial, sans-serif;
}
}
@media print {
h1 {
font-size: 11px;
font-family: Times, sans-serif;
}
}
/* This style block applies to all medias */
h2 {
font-size: 10px;
}
You can also specify the media with the @import
statetement, at the beginning of the file
@import url("css/printer.css") print;
@import url("css/browser.css") screen;
or with <link>
tags in html:
<link rel="stylesheet" media="print" href="css/printer.css">
Units
Many CSS properties use length values which must be specified with a number and a unit.
The length can be expressed with absolute or relative values.
Note: Whitespaces between the number and the unit are forbidden.
Absolute
Absolute values will be applied whatever media is rendering the page. As a consequence, if the page was designed for desktop, it won't be rendered beautifully on mobile devices since all dimensions are hard-coded and were specified for bigger screens. For scalibility, always prefer relative lengths.
p {
/* You can pick any unit from the followings:
* 'in': inches (~2.54cm)
* 'cm': centimeters
* 'mm': millimeters
* 'pt': point=1/72 inch (~0.35mm)
* 'pc': picas=12 pt
* 'px': pixel=0.75pt
* For on-screen display, consider using 'px' only as the others are better for
* print media or other high resolution devices. */
margin: 12px;
}
Relative
Relative length units are ratios of an absolute dimension.
p {
font-size: 10px;
/* You can pick any unit from the followings:
* 'em': ratio of current font-size
* 'ex': ratio of the height of letter 'x' in current font
* 'rem': ratio of root's font size
* 'vh' : 1% of viewport's height
* 'vw' : 1% of viewport's width
* '%' : 1% of parent's property */
padding: 1.2em; /* 12 px */
}
Note that font-size
could have been specified with relative units as well. In that case, the font-size
of the parent element would be used, and if relative as well, its parent and so on until reaching the body's default which is 16px.