CSS: Elements styling
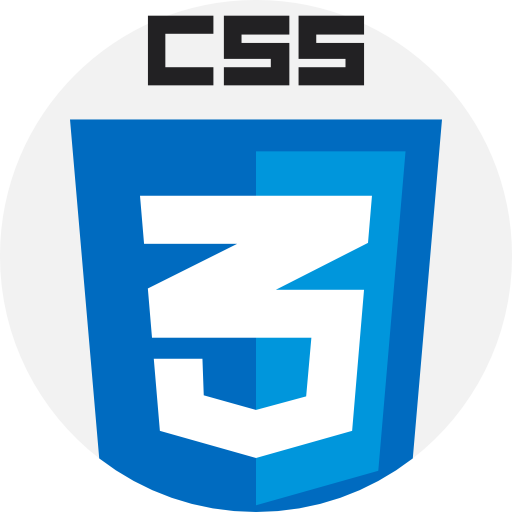
Here are some element-specific styling properties that you will necessarily use at some point.
Text
h2 {
/* Text color */
color: #5470f0;
/* Horizontal alignment (left, right, center, justified).
* 'justify' stretches each line so that they all have equal width
* except for the last one. */
text-align: center;
/* Add a line under, on or on top of the text (underline, line-through, overline, none).
* Tip: used 'none' with 'a' to remove the default underline for links*/
text-decoration: 14px;
/* Set the case (uppercase, lowercase, capitalize.
* 'capitalize' sets the first letter of each word to uppercase */
text-transform: uppercase;
/* Indent the first line or a paragraph (%, px or any length value) */
text-indent: 10px;
/* Customize space between characters (px or any length value)*/
letter-spacing: -3px;
/* Customize space between words (px or any length value)
* Note: If alignment is set to 'justify', word-spacing is affected. */
word-spacing: 2px;
/* Set inter-lines spacing (%, px or any length value) */
line-height: 1.2; /* when setting a number, you set a ratio of the font size */
/** CSS3 **/
/* Configure how overflowing text (e.g. container too small) should be rendered.
* 'clip': overflowing text is clipped and becomes invisible
* 'ellipsis': displays '...' at the end of the line
* string value: displays the provided string value at the end of the line */
text-overflow: ellipsis;
/* Define wether the long words at the end of the line should overflow ('normal') or be wrapped
* onto next line ('break-word') */
word-wrap: break-word;
/* Define how words with '-' character should be displayed when they are too long for the line.
* 'break-all': the entire word is wrapped onto next line
* 'keep-all': the word is cut on '-' character and the non-fitting part is wrapped */
word-break: keep-all;
}
Links
The default rendering of links can be easily edited thanks to pseudo-class selectors fo a
:
:link
: univisted link:visited
: visited link:hover
: mouse over link:active
: active link
a:link { /* unvisited link */
color: #ff0000;
text-decoration: none;
border-bottom: 1px solid;
}
a:visited { /* visited link */
color: #ff00ff;
}
a:hover { /* mouse over link */
color: #00ff00;
border-bottom: none;
}
a:active { /* active link */
color: #00ffff;
}
Lists
The following styles apply to all types of list (ul
, ol
, dl
).
/* The next styles could also be applied to 'ul li' */
ul {
/* Change default marker (bullet or number) type.
* (disc (default), circle, square, upper-roman, lower-latin,...)
https://developer.mozilla.org/fr/docs/Web/CSS/list-style-type#disc
*/
list-style-type: square;
/* Set the position of the marker to be inside text boxes or
* outside (default) */
list-style-position: inside;
/* Use an external image for the bullet */
list-style-image: url("images/bullet.png");
}
Shorthand
A less verbose property can be used to set all properties at once.
ul {
/* Shorthand notation: type position image */
list-style: square inside url("images/bullet.png");
}
Tables
By default, tables are rendering without borders. CSS offers several styling enhancements.
table, th, td {
/* Define border : thickness type color */
border: 1px solid black;
/* Since each table element (table, th, td) has its own border
* you get duplicated lines by simply setting a border. You can
* collapse these lines so that there is no duplication anymore
* with this option. Values are 'collapse' and 'separate' (default) */
border-collapse: collapse;
/* Only applicable if collapse is set to 'separate', this property
* sets the space between the borders of the cells. In other conditions
* simply use the 'padding' property */
border-spacing: 10px;
/* Only applicable if collapse is set to 'separate', this property
* configure the behavior of the view with empty cells. By default
* their border is drawn (show) but it can be hidden (hide) */
empty-cells: hide;
/* By default, the table will adapt the column width to their content.
* You can override this behavior by telling it to distribute the space
* evenly among columns for a fixed size.
* Values: auto (default), fixed (limited but faster rendering) */
table-layout: fixed;
width: 400px;
/* Set horizontal text alignment in rows.
* By default, td is left-aligned while th is centered */
text-align: right;
/* Set vertical text alignment in rows.
* By default, the alignment is middle. */
vertical-align: bottom;
}
/* Zebra striped table. Every odd row of the table will have a darker
* background color thanks to the pseudo-class slector 'nth-child.
* Note that the style is only applied to rows of html5's tbody element
* so that headers are not included */
tbody tr:nth-child(odd) {
background-color: #f2f2f2;
}
caption {
/* Positionning of the caption (top, bottom).
* Use text-align to align left or right.*/
caption-side: bottom;
}
Responsiveness
By default, tables are not responsive. To add scrolling support, you need to wrap the table in a div
and apply the style overflow-x: auto
to it as shown below.
<div style="overflow-x: auto;">
<table>
... table content ...
</table>
</div>
Cursors
The cursor is the mouse pointer displayed when you navigate through a website. Many html elements define their own, such as links or inputs, which is why the cursors changes when you hover them. With cursor
you can change the displayed cursor or define your own with .cur
(default standard), .gif
, .png
or .jpg
images (in recent browsers only).
a {
/* There are many built-in cursors (default, pointer, text, wait, progress, crosshair, move,...)
* and you can also use images to display custom ones.
* When you select several cursors, make sure to set a valid one at the end so that there is
* always a valid fallback value */
cursor: url("custom.gif"), crosshair;
}
Overflow
overflow
(and overflow-x
, overflow-y
) define how the content of an element should be displayed when it is bigger than its content area (e.g. when height
and width
are too small).
div {
height: 50px;
width: 100px;
/* Define how the value will be rendered.
* 'visible' (default) : content rendered outside of its box and overlaps other elements
* 'hidden': content clipped and extra data not visible
* 'scroll': content clipped but scrollbars let see the hidden parts
* 'auto': scrollbars are created if content is too big */
overflow: auto;
}