HTML fundamentals
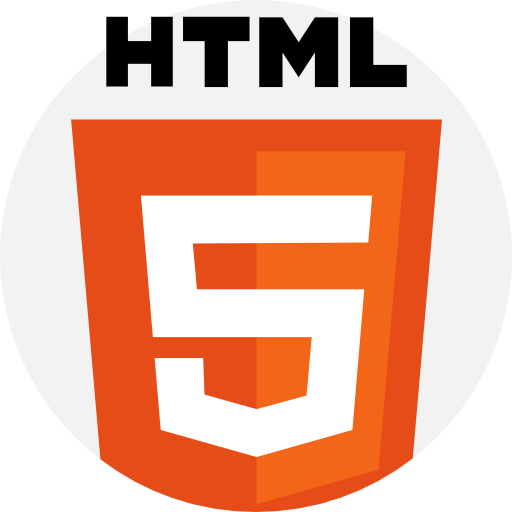
Welcome to the HTML Fundamentals article, where we'll explore key HTML elements and concepts that form the foundation of web pages. Whether you're a seasoned developer or just starting your journey, this series aims to provide valuable insights into HTML, the markup language that structures the content of the World Wide Web.
Each chapter provides concise explanations, code snippets, and practical examples to empower you with a solid understanding of HTML fundamentals.
Head
The head elements collectively describe the properties of the document (title, character set, stylesheets, scripts).
<head>
<!-- Mandatory: the title should ideally be smaller than 65 chars -->
<!-- to be indexed properly by search engines -->
<title>My fantastic website</title>
<!-- You can add more data about your website in meta tags that'll be -->
<!-- used by browsers or search engines -->
<meta charset="utf-8">
<meta name="author" content="John Smith">
<meta name="description" content="Presentation of different IoT projects built with Arduino">
<!-- Viewport helps the rendering of your page on mobile devices. You -->
<!-- should ideally always have a viewport defined to avoid pinching -->
<!-- and zooming to read its content. Here, the width is defined to be -->
<!-- limited to the device-width, at 100% -->
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- You can define the base address for all relative -->
<!-- links in the document -->
<base href="https://mywebsite.com/">
<!-- You can refer to CSS stylsheets -->
<link rel="stylesheet" href="style.css">
<!-- or embed styles -->
<style>
body { background-color: YellowGreen; }
h1 { color: red; }
p { color: green; }
</style>
<!-- You can refer to javascript files -->
<script src="coloring-utils.js"></script>
<!-- or embed javascript -->
<!-- Note: Ideally, the script tags should be placed at the bottom -->
<!-- of the html, in the 'body' section to speed up the rendering of the page -->
<script>
document.write("<h1>Hello World!</h1>")
</script>
</head>
Page layout
HTML5 introduced new tags to better structure a web page and no longer rely only on div
tags:
<header>
: header of a document or a section<footer>
: footer of a document or a section<nav>
: section with navigation links<section>
: represents a section of a document<article>
: self-contained unit of information (such as a blog post)<aside>
: content losely related to the page content (such as a section for ads)
Here is how these tags can be applied to a section or a document:
----------------------------------------
header
----------------------------------------
| |
Nav | Section | Aside
| |
| -------------------- |--------
| | Article | |
| | | |
| -------------------- |
----------------------------------------
footer
----------------------------------------
And here is an example of layout in HTML5:
<div class="container">
<header>
<h1>Sports news</h1>
</header>
<div class="wrapper">
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">Soccer</a></li>
<li><a href="#">Basketball</a></li>
</ul>
</nav>
<section>
<h2>Lakers failed again</h2>
<p>The desire of success was not...</p>
</section>
</div>
<footer>
<p>copyright © sports news</p>
</footer>
</div>
Note that depending on how you want to wrap elements or how you want to style them, use div
tags to create groups.
HTML Element types
There are two groups of elements:
- block level: used to structure the page (
div
,p
,h1-h6
,form
,ul
,ol
, ...). They start by a line break, end with a line break and expand on full width. - inline level: used to fill the content (
img
,a
,span
,strong
,b
,input
,button
...). They are inserted in place and just take the required space.
Note that the default display type of an element can be overriden with css (display
).
Empty elements
Some elements don't need closing tags. Here is an example with <br>
<p>This is <br> great</p>
Note that <br>
is different than self-closing tags as it does not end with />
. This if fine for HTML, only xHTML always requires />
.
Attributes
General purpose
Some attributes are valid for all HTML elements : id
, title
, class
, style
.
Boolean attributes
Several attributes do not consist of name/value pairs but just of a name, such as checked
, disabled
, readonly
... The shortened syntax is equivalent to checked="true"
for instance.
Headings
h1
to h6
.
Don't use them to display big or bold text. Instead, use them to structure your documents as search engines index the structure and the content of the pages.
Paragraphs
Use <p>
(paragraph) to publish text on a page. Note that any space or line breaks in the text written within <p>
is not taken in consideration when rendering.
<p>The spaces and line
breaks of this paragraph won't show
at rendering. Instead, you'll se a single line with no spaces
</p>
You can combine <p>
with the following tags to customize the output:
<br>
: line break<hr>
: horizontal delimitation with a line between paragraphs
: special character (here non-breaking space)
<p>The spaces ` and line <br> breaks of this paragraph</p>
<hr>
<p>will show at rendering</p>
Alternatively, consider <pre>
to render a text exactly like it's written:
<pre>The spaces and line
breaks of this paragraph will show
at rendering.
</pre>
Text formatting
<p>This is <b>bold text</b>.</p>
<p>This is <strong>strongly important text</strong>.</p>
<p>This is <i>italic text</i>.</p>
<p>This is <em>emphasized text</em>.</p>
<p>This is <mark>highlighted text</mark>.</p>
<p>This is <code>computer code</code>.</p>
<p>This is <small>smaller text</small>.</p>
<p>This is <sub>subscript</sub> and <sup>superscript</sup> text.</p>
<p>This is <del>deleted text</del>.</p>
<p>This is <ins>inserted text</ins>.</p>
<i>
vs <em>
and <b>
vs <strong>
Both are used to format a text in italic or bold respectively. The only difference is that em
emphasizes that its content is important while i
is pure styling.
Same goes for b
and strong
where b
is pure styling while strong
stresses the importanced of its content.
Quotations
Use <blockquote>
to auto-indent a qutotation block that comes from other sources.
<blockquote>
<p>This text is an extract of an external resources</p>
<cite>- M. Author</cite>
</blockquote>
<cite>
is used to describe a reference to a creative work. It must include the author name or the title of the work or eventually an URL.
Inline quotations can also be inserted by using <q>
<p>As once said by a wise man, <q>live your dreams</q></p>
Note that in this case, the text will simply be surrounded with double quotes "
.
Abbreviations
Not everyone knows all the abbreviations that you could use in your text. <abbr>
solves this problem by attaching a description to an abbreviation that the user can see by hovering over it.
<p>The <abbr title="United Nations">UN</abbr> decided to not take action</p>
The abbreviation will have a dotted underline to catch user's attention.
Address
HTML provides a special tag to encapsulate a contact information.
<address>
Nico Burger<br>
7 Brooklyn Avenue<br>
London
</address>
Images
Images can be inserted with the inline and empty element <img>
.
<p>A drawing made by an artist <img src="url" alt="Artistic drawing"></p>
alt
provides an alternative text for the image if it cannot be displayed.
<img>
lets you set the image dimensions with height
and weight
attributes but you can also assign those in the CSS stylesheet.
Picture
<img>
comes with several limitations:
- scaling the image to fit different devices is difficult
- changing
height
andwidth
attributes does not reduce the file size
HTML5 introduced a new tag <picture>
to accomodate these limitations.
<picture>
<source media="(min-width: 1000px)" srcset="large.png">
<source media="(min-width: 500px)" srcset="medium.png">
<img src ="default.png" alt="Company">
</picture>
<picture>
works like a switch/case
. The browser evaluates the best match among the specified source
tags and uses the default <img>
tag if none matches.
Note that the default <img>
tag is mandatory.
Image maps
As often used on facebook, your image can contain clickable links on its surface. This is possible with <map>
:
<img src="earth_landscape.png" usemap="#capitals" alt="Capitals on earth">
<map name="capitals">
<area shape="circle" coords="127, 54, 654" href="paris.html" alt="Paris">
<area shape="circle" coords="475, 99, 300, 147, 45, 98" href="beyrouth.html" alt="Beyrouth">
</map>
<img>
references the map by its name
. Each area
inside the map defines a clickable link.
Since creating these maps manually is not convenient, you could consider using one of the numerous online tools.
Note that <map>
is not search-engine-friendly.
Tables
Use tables to display tubular data only (never use them to build the layout of a page, they're slow to render and hard to maintain).
A <table>
is made of rows <tr>
, columns <td>
and can eventually contain a caption caption
and a header <th>
.
<table>
<caption>Users Info</caption>
<tr>
<th>Name</th>
<th colspan="2">Phone</th>
</tr>
<tr>
<td>John Carter</td>
<td>5550192</td>
<td>5550152</td>
</tr>
</table>
In the example above, the header is horizontal (along a row) and we're making use of the colspan
attribute to span the header Phone
on 2 columns.
Here is another example with a vertical header and the use of rowspan
to span the header on 2 rows:
<table>
<tr>
<th>Name:</th>
<td>John Carter</td>
</tr>
<tr>
<th rowspan="2">Phone:</th>
<td>55577854</td>
</tr>
<tr>
<td>55577855</td>
</tr>
</table>
Finally, not that HTML5 introduced additional tags to add more structure into a table woth <thead>
, <tbody>
and tfoot
.
<table>
<thead>
<tr>
<th>Items</th>
<th>Expenditure</th>
</tr>
</thead>
<tbody>
<tr>
<td>Stationary</td>
<td>2,000</td>
</tr>
<tr>
<td>Furniture</td>
<td>10,000</td>
</tr>
</tbody>
<tfoot>
<tr>
<th>Total</th>
<td>12,000</td>
</tr>
</tfoot>
</table>
Lists
Unordered
The displayed elements are marked with a bullet.
<ul>
<li>Butter</li>
<li>Milk</li>
<li>Sugar</li>
</ul>
Ordered
The displayed elements are marked with a number.
<ol start="5">
<li>Open the box</li>
<li>Replace the battery</li>
<li>Close the box</li>
</ol>
By default, start
attribute equals 1
which the starting number for the ordering. However, you can set it to other values if needed.
Description
A description list <dl>
displays items <dt>
with a definition or description <dd>
for each. The descriptive text is usually automatically indented by browsers.
<dl>
<dt>Bread</dt>
<dd>A baked food made of flour.</dd>
<dt>Coffee</dt>
<dd>A drink made from roasted coffee beans.</dd>
</dl>
Forms
Forms are used to collect user inputs and submit them.
<form name="uniqueName" action="url" method="post" target="_self">
<label>Name: <input type="text"></label>
<input type="submit" value="Submit">
</form>
<form>
accepts several types of attributes:
name
: name of the form. Must be unique in the document and not emptyaction
: url of program or script that will process the inputsmethod
: http verb used to send the data :post
(default) orget
(means that the data is visible in browser's address bar)target
: specifies where the response should be displayed (_blank
,_self
,_parent
,_top
)
A form can contain several type of controls depending on what it does.
Inputs
Most commonly used element, the input can take different forms depending on its type
attribute.
<form>
<!-- Text field -->
<!-- Note that label and input are separate here. 'for' and 'id' -->
<!-- are used to join the two. The condensed syntax shown above is also possible -->
<label for="user-name">Username:</label>
<input type="text" name="username" id="user-name">
<!-- Text area field -->
<!-- The input here is multiline -->
<label for="comment-area">Comment:</label>
<textarea type="text" name="comment" id="comment-area"></textarea>
<!-- Password field -->
<!-- The input here is masked with dots -->
<label for="user-pwd">Password:</label>
<input type="password" name="user-password" id="user-pwd">
<!-- Radio buttons field -->
<!-- Note tha the 'name' is identical for each input to group them together. -->
<!-- You can only select one item per group. -->
<input type="radio" name="gender" id="male">
<label for="male">Male</label>
<input type="radio" name="gender" id="female">
<label for="female">Female</label>
<!-- Checkboxes field -->
<!-- You can only select any item per group. -->
<!-- Here, the attribute 'checked' sets the default state of a checkbox. -->
<!-- Same attribute is valid for radio buttons -->
<input type="checkbox" name="options" id="opt-dark" checked>
<label for="opt-dark">Use dark mode</label>
<input type="checkbox" name="options" id="opt-hour">
<label for="opt-hour">Use 24h mode</label>
<!-- File select field -->
<!-- Renders a button that will open a file explorer -->
<label for="file-select">Upload:</label>
<input type="file" name="upload" id="file-select">
<!-- Select boxes field -->
<!-- Renders a combobox with different options -->
<label for="city">City:</label>
<select name="city" id="city">
<option value="sydney">Sydney</option>
<option value="melbourne">Melbourne</option>
<option value="cromwell">Cromwell</option>
</select>
<!-- Submit button -->
<!-- Triggers the processing of submitted data -->
<!-- Note that you can also use a 'button' here for more -->
<!-- rendering options -->
<input type="submit" value="Submit">
<!-- Reset button -->
<!-- Clears user inputs -->
<input type="reset" value="Reset">
</form>
There are other inputs as well for colors, email, datetime, number, range, search, phone number, url ...
Groups
You can add more structure to your forms by grouping some inputs together in named panels with <fieldset>
and legend
.
<form>
<fieldset>
<legend>Name</legend>
<label>Firstname: <input type="text" name="firstname"></label>
<label>Lastname: <input type="text" name="lastname"></label>
</fieldset>
<fieldset>
<legend>Contact Details</legend>
<label>Email Address: <input type="email" name="email"></label>
<label>Phone Number: <input type="text" name="phone"></label>
</fieldset>
</form>
iFrame
<iframe>
lets you display an external html page inside yours. It pretty much acts like a mini web browser within a web browser.
<iframe src="hello.html" width="400" height="200"></iframe>
Note: height
and width
can be expressed in %
as well.
The iFrame can also be used to render a link accessed within the page thanks to target
and name
attributes.
<iframe src="weather.html" name="weatherFrame"></iframe>
<p><a href="http://myweather.org/city=paris" target="weatherFrame">Show weather in Paris</a></p>
The external link will be rendered inside of the iFrame.
Canvas
HTML5 only.
The canvas is a two-dimensional rectangular area that can be used to draw graphics on the webpage via Javascript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Drawing on Canvas</title>
<script>
window.onload = function() {
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
// draw a line
context.moveTo(50, 150);
context.lineTo(250, 50);
context.stroke();
};
</script>
</head>
<body>
<canvas id="myCanvas" width="300" height="200"></canvas>
</body>
</html>
You can draw many shapes and colors in a canvas. The content of a canvas can be saved into a file.
SVG
HTML5 only.
An SVG is an XML-based image format that can be scaled without any loss of quality. The SVG also supports animations and can be edited dynamically with Javascript.
<svg width="300" height="200">
<!-- Example of a line-->
<line x1="50" y1="50" x2="250" y2="150" style="stroke:red; stroke-width:3;" />
<!-- Example of a rectangle-->
<rect x="50" y="50" width="200" height="100" style="fill:orange; stroke:black; stroke-width:3;" />
<!-- Example of a circle -->
<circle cx="150" cy="100" r="70" style="fill:lime; stroke:black; stroke-width:3;" />
<!-- Example of text -->
<text x="20" y="30" style="fill:purple; font-size:22px;">
Welcome to Our Website!
</text>
</svg>
Audio
HTML5 only.
<audio>
element lets you embed sound in web pages.
<audio controls="controls">
<!-- You can defined many sources for the same sound -->
<!-- and the browser will pick the first it supports -->
<source src="media/birds.mp3" type="audio/mpeg">
<source src="media/birds.ogg" type="audio/ogg">
</audio>
Video
HTML5 only.
<audio>
element lets you embed videos in web pages.
<video controls="controls">
<!-- You can defined many sources for the same video -->
<!-- and the browser will pick the first it supports -->
<source src="media/shuttle.mp4" type="video/mp4">
<source src="media/shuttle.ogv" type="video/ogg">
</video>
Web storage
HTML5 only.
HTML5's we storage provides you with a solution to store 5MB of data locally on computer whereas a cookie was only capable of storing 4KB and had the inconvenient of being transferred on each request.
There are 2 types of storages with different scope and lifetime as explained below.
Local storage
Stores data permanently for an entire website.
<script>
// Store data
localStorage.setItem("first_name", "Peter");
// Retrieve data
console.log("Hi, " + localStorage.getItem("first_name"));
</script>
Session storage
Stores data temporarily for a single browser tab and deletes the data when the browser or tab is closed.
<script>
// Store data
sessionStorage.setItem("first_name", "Peter");
// Retrieve data
console.log("Hi, " + sessionStorage.getItem("first_name"));
</script>
Application cache
HTML5 only.
Caching helps with performances as your browser will store some of the resources accessed for a web page and load them back from storage instead of requesting them againg from the server. In HTML5, a caching manifest can be specified on server side to tell the browser what to cache:
CACHE MANIFEST
# v1.0 : 10-08-2014
# All the items under CACHE: will be cached
CACHE:
# pages
index.html
# styles & scripts
css/theme.css
js/jquery.min.js
js/default.js
# images
/favicon.ico
images/logo.png
# All the items under CACHE: will not be cached
# meaning that the access to login.php is only
# possible if the user is online
NETWORK:
login.php
# Fallback URIs. The index of the page will be redirected
# to offline.html when the user is offline
FALLBACK:
/ /offline.html
The cache manifest needs to be indicated in the html
element:
<!DOCTYPE html>
<html lang="en" manifest="mywebsite.appcache">
Web workers
HTML5 only.
The execution of javascript in the web browser is single threaded by default, meaning that any pending execution will prevent the user from interacting with the page.
For intensive computations that can be executed in background, HTML5 has introduced web workers to keep the page responsive.
To use this feature, you must first create a javascript file which will contain the code to be executed in background. Note that this code cannot access the DOM.
// worker.js
var i = 0;
function countNumbers() {
if(i < 100000) {
i = i + 1;
postMessage(i);
}
// Wait for sometime before running this script again
setTimeout("countNumbers()", 500);
}
countNumbers();
Now from the web page, you can control the execution of the worker and/or consume its result as shown below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Using Web Worker</title>
<script>
// You can control the execution from the web page
// Here is how you can start the execution
function startWorker() {
// Create a new web worker
var worker = new Worker("worker.js");
// Everytime the worker calls postMessage, this function will be executed
worker.onmessage = function(event) {
document.getElementById("result").innerHTML = event.data;
}
worker.postMessage("start");
}
// Here is how you can stop the execution
function stopWorker() {
worker.terminate();
}
</script>
</head>
<body>
<h1>Web Worker Demo</h1>
<button type="button" onclick="startWorker();">Start web worker</button>
<button type="button" onclick="stopWorker();">Stop web worker</button>
<div id="result">
<!--Received messages will be inserted here-->
</div>
</body>
</html>
Server sent events
HTML5 only.
When a client communicates with a server, the connection is closed after receiving the response which is sufficient most of the time but does not cover the situations where data needs to be streamed.
In HTML5, a web page can hold a connection open so that the web server can send new responses automatically at any time. Server-Side Events (or SSE) are unidirectional responses delivered from server to client.
// server_time.php
<?php
// On server side we set the content-type to text/event-stream
// to enable SSE
header("Content-Type: text/event-stream");
// We disable caching to prevent the browser from storing the data
header("Cache-Control: no-cache");
// Get the current time on server
$currentTime = date("h:i:s", time());
// Send it in a message
// The SSE sent by the server must start with 'data:'
echo "data: " . $currentTime . "\n\n";
flush();
?>
On client side, we simply attach an event handler to the EventSource
object.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Using Server-Sent Events</title>
<script>
window.onload = function() {
var source = new EventSource("server_time.php");
source.onmessage = function(event) {
document.getElementById("result").innerHTML += "New time received from web server: " + event.data + "<br>";
};
};
</script>
</head>
<body>
<div id="result">
<!--Server response will be inserted here-->
</div>
</body>
</html>