Javascript : arrays
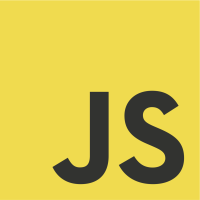
Second article in my javascript series and this one is dedicated to arrays. As a daily user of LINQ expressions in .NET, I needed to know how similar things can be tackled in javascript. As usual, this article is brief and essentially shows documented code.
// An array is an 'object' in javascript
// Arrays can mix types
let colors = ['red', 'green', 0, 1];
// The size of an array is dynamic
colors[4] = 'purple';
console.log(colors.length); // prints 5
// Warning, adding items with high indexes creates 'undefined' holes in the array
colors[6] = 'pink'; // ['red', 'green', 0, 1, 'purple', undefined, 'pink']
// Sort the array
colors.sort(); // [0, 1, 'green', 'pink', 'purple', 'red', undefined]
// Reverse the array
colors.reverse();
You can't access array elements with named indexes. If you try, your variable will be converted to an object
.
Tip: any object can be converted to an array with Object.values(x)
. This is useful to print the properties of an object easily:
let person = {
name: "John",
age: 41,
size: "5'2",
};
console.log(Object.values(person)); // ['John', 41, "5'2"]
Basic operations
// Get length
let length = colors.length;
// Append data
colors.push('blue');
// Remove last data
colors.pop(); // 'blue' removed
// Prepend data
colors.unshift('red'); // 'red' insert at first position
// Remove first data
colors.shift(); // 'red' removed
// Remove 1 element
colors.splice(/*index*/ 1, /*quantity*/ 1); // 'green' removed
// Insert 1 element
colors.splice(/*index*/ 1, /*quantity*/ 0, 'green'); // 'green' reinserted
// Get slice of array
let slice = colors.slice(/*index*/ 1, /*quantity*/ 1); // slice = ['green', 0]
// Concatenate 2 arrays
let concat = colors.concat(['yellow', 'grey']); //concat=['red', 'green', 0, 1, 'yellow', 'grey']
// Avoid using 'delete' as it leaves 'undefined' values in the array
colors.delete('green'); // ['red', undefined, 0, 1]
// https://www.w3schools.com/jsref/jsref_obj_array.asp
map()
Equivalent of Select
in C#, map()
creates a new array by applying a given function to each element.
const input = [1, 2, 3];
const output = input.map((num) => num * num); // [1, 4, 9]
filter()
Equivalent of Where
in C#, filter()
creates a new array by applying a selection predicate to each element.
const input = [1, 2, 3, 4, 5];
const output = input.filter((num) => num >= 3); // [3, 4, 5]
reduce()
Equivalent of Aggregate
in C#, reduce()
reduces an array to s ingle value by applying a formula and accumulating the result.
const input = [1, 2, 3, 4, 5];
const output = input.reduce((acu, current) => acu + current); // 15
forEach()
Execute a function with each element of an array.
const input = [1, 2, 3, 4, 5];
const output = input.forEach((num) => console.log(num)); // prints each element on a single line
find()
Equivalent of FirstOrDefault
, find fetches the first element that satisfies a condition (or undefined
).
const input = [
{ id: 1, age: 25 },
{ id: 2, age: 35 },
{ id: 3, age: 40 },
];
const output = input.find((i) => i.age < 40); // { id: 1, age: 25}
every()
Equivalent of All
, checks if all elements of an array satisfy a condition.
const input = [1, 2, 3, 4, 5];
const output = input.every((i) => typeof i == 'number'); // true
some()
Equivalent of Any
, checks if any elements of an array satisfies a condition.
const input = [1, 2, 3, 4, 5];
const output = input.some((i) => i == 6); // false