Javascript : Fundamentals
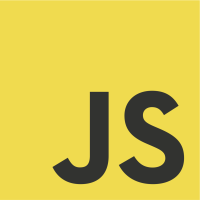
After 15 years of coding experience with languages like C#, C/C++ and python, i wanted to step into javascript. This is the first article of a series that will give you the basics to start working with this language smoothly. The article is mostly written with code examples and rare text explanations so that it is easy to grasp for any developer. Some obvious details are omitted when they are common to almost all programming languages.
Introduction
Javascript is the most popular programming language.
It was thought to run in internet browsers where an engine executes the code (SpiderMonkey
on firefox, v8
on Chrome).
Later came an engine that lets you run javascript code on a computer with Node.js
. So javascript can be used for front-end as well as backend developments.
ECMA is a company defining standards. It defined ECMAScript which is the specification to which Javascript conforms.
The original specification was released in 1997 (v1
) and later came a new version in 2015 called ES2015
or ES6
. Subsequent versions were released but ES6
can be considered as the new standard.
Running javascript in a browser
Create 2 files : index.html
and index.js
in the same folder.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello World</h1>
<script src="index.js"></script>
</body>
</html>
console.log('Hello World');
Now open index.html
in your browser and press F12
to open developer tools. Select Console
and you should see Hello world
printed.
Running javascript in node
Prerequisite : install Node.js.
Open a terminal in the folder containing the previous two files and type
node index.js
You should see Hello World
printed in the console.
Variables
// Variables are case sensitive
// ES6 legacy
// Best practice: never use var (more on that further below)
var myVariable1; // by default = undefined
// New way
let myVariable2 = 'Turbo';
// Cannot be reserved keyword
// let if, else, for, ...;
// Cannot start with a number
// let 1tool, 2things, ...;
// Cannot space or hyphen (-)
// let my-tool, your bread;
Constants
const interestRate = 0.3; // immutable
// Don't get confused: a const reference type is mutable
const array = [0, 1, 2];
array[0] = 1; // works
Primitive types
// Primitives (value types)
// String
// string are iterable
let name = 'Cem';
console.log(typeof name); // prints 'string'
// Number
let age = 30;
console.log(typeof age); // prints 'number'
// Boolean
// Everything with a value is true
// but 0, -0, undefined, null, NaN are interpreted as false
let isAdult = true;
console.log(typeof isAdult); // prints 'boolean'
// Undefined
let religion = undefined; // or just let religion;
console.log(typeof religion); // prints 'undefined'
// Null
let car = null;
console.log(typeof car); // prints 'object'. See further below
Cast
// string to number
let n = Number("1"); // n=1
// Simpler: use the unary operator
let n = + "1"; // n=1
Bigint
An integer can only be represented up to 15 digits. Beyond that limit, you need to append n
to your number to use the bigint
type internally.
let bigint = 1234567890123456n;
NaN
NaN
is a special number value, meaning Not A Number
.
It is the value returned by invalid operations:
let a = + "n"; // return NaN because you can't cast n to a number
let b = 1 / "n"; // return NaN because you can't divide by a string
Dynamic typing
The type of a variable is determined at runtime, depending on the value it contains. Variable can mutate if they are assigned to a different data type.
Objects
// Use {} to declare an object literal
let person = {
name: 'Cem',
age: 30
};
// Change a property value
// dot notation
person.age = 31;
// bracket notation
person['age'] = 31;
Comparison operators
// The following are similar to other languages
// >, <, >=, <=
// Equality comparer, don't be fooled
console.log(8 == 8); // true
console.log(8 == '8'); // true
console.log(8 === '8'); // false
There is a major difference between ==
and ===
. The former casts the operands to the same type before doing the comparison whereas the latter compares the type and the value. Same logic for !==
and !===
.
if/else
Identical to other languages.
if(x > y){
} else if(x > z){
} else {
}
switch / case
Identical to other languages.
switch(expression) {
case x:
// code block
break;
case y:
case z:
// code block
break;
default:
// code block
// break is optional here
}
Loops
for / while / do,while
Identical to other languages.
// for loop
for (let i = 0; i < cars.length; i++) {
text += cars[i] + "<br>";
}
// while loop
let i = 0;
while (i < cars.length){
text += cars[i] + "<br>";
i++;
}
// do while loop
do {
// code block to be executed
}
while (condition);
for in
Don't get confused : for in
iterates over the properties of an object, not the values.
const person = {fname:"John", lname:"Doe", age:25};
let text = "";
for(let x in person) {
console.log(x); // prints 'fname', 'lname', 'age'
text += person[x];
}
console.log(text);// prints 'John Doe 25'
for of
for of
iterates over the values of an object.
const numbers = [45, 4, 9, 16, 25];
for(let x of numbers) {
console.log(x); // prints 45, 4, 9,...
}
break / continue
Similarly to other languages, you can control the loop flow with break
to exit or continue
to jump to next iteration.
Functions
function sayHello(text) {
console.log('Hello '+ text);
}
function square(number) {
return number * number;
}
sayHello('Cem');
let number = square(2);
Arrow functions
Introduced with ES6.
let myFunction = (a, b) => a * b;
Strict mode
"use strict"; // used to write 'secure' javascript
// from here on, no more undeclared variables
x = 3; // will throw an error
// other limitations as explained here https://www.w3schools.com/js/js_strict.asp